Blog
Ontdek de nieuwste trends en ontwikkelingen op het gebied van Identity & Access Management en vind inspirerende inzichten en expertise.
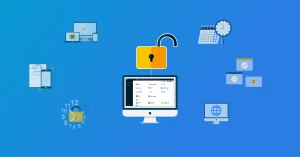
- Product en technologie
Access Management – Toegang op basis van context
1 juli 2025
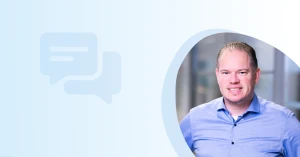
- Product en technologie
Accountmanager, jouw directe lijn met Tools4ever
25 juni 2025
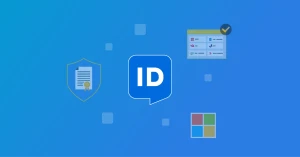
- Product en technologie
Access Management – Opzetten van applicatie-integratie middels SAML, WS-Federation, OpenID Connect
17 juni 2025
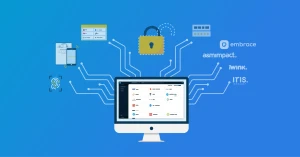
- Product en technologie
Access Management – Integratie van dashboard in bestaand intranet
10 juni 2025
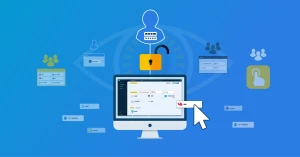
- Product en technologie
Access Management – Dashboard, categorieën en toegangsrechten configureren
26 mei 2025
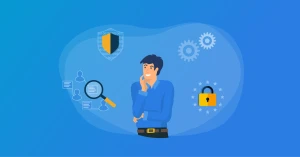
- Security en compliance
Het belang van governance bij IAM
19 mei 2025
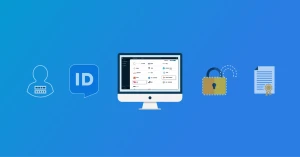
- Product en technologie
Access Management – Configureren van SSO via applicatiecatalogus
8 mei 2025
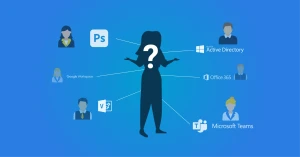
- Security en compliance
Nog niet IAM compliant?
23 april 2025
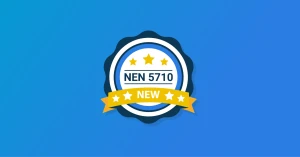
- Nieuws en updates
Nieuwe NEN 7510:2024 beschikbaar
14 april 2025
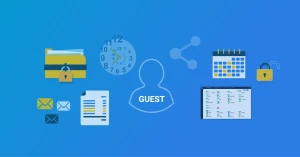
- Product en technologie
Access Management – Gasttoegang beheren
25 maart 2025
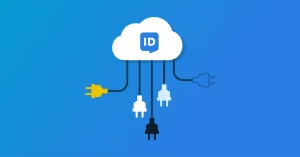
- Product en technologie
Access Management - Een of meerdere Identity Providers koppelen
10 maart 2025
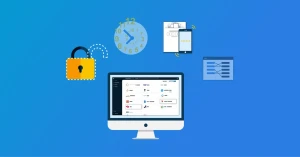
- Product en technologie
Access Management - Waar te starten?
19 februari 2025
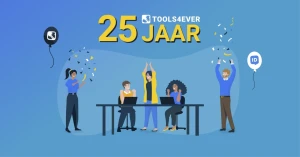
- Nieuws en updates
Het verhaal achter 25 jaar Tools4ever
17 februari 2025
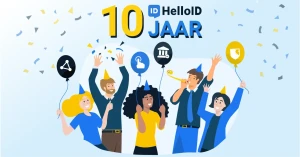
- Product en technologie
HelloID bestaat 10 jaar!
23 januari 2025
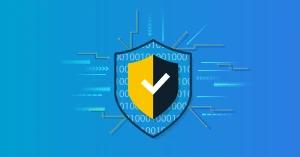
- Nieuws en updates
Cybersecurity, klaar voor 2025?
16 januari 2025
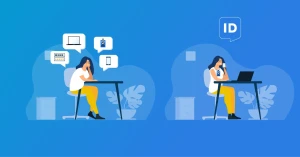
- Industrie en trends
Geef nieuwe medewerkers een vlotte start
8 januari 2025
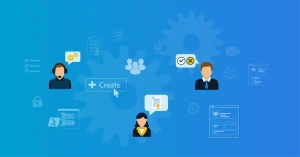
- Product en technologie
Service Automation – Adoptie
4 december 2024
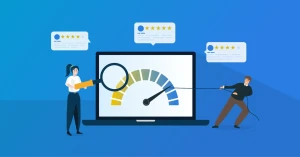
- Nieuws en updates
Klanten waarderen Tools4ever met een 8,4
2 december 2024
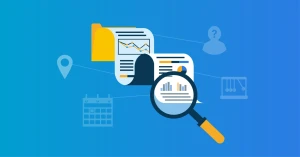
- Product en technologie
Service Automation – Auditing
25 november 2024
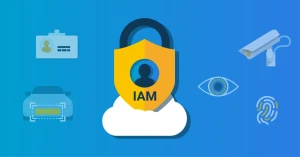
- Product en technologie
Alle toegang beheren met HelloID?
6 november 2024
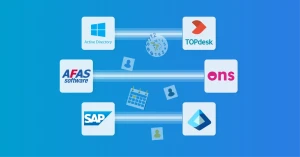
- Product en technologie
Rechtstreekse koppeling voor je accountbeheer?
31 oktober 2024
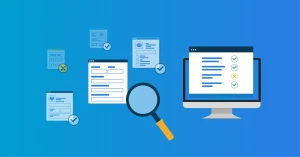
- Product en technologie
Service Automation - Testen en Logging
28 oktober 2024
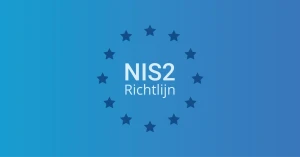
- Nieuws en updates
NIS2 nu gepland in Q3 2025
24 oktober 2024
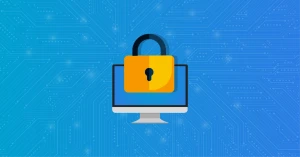
- Industrie en trends
Slimmere informatie- en toegangsbeveiliging met AI
14 oktober 2024
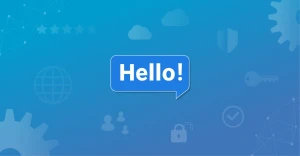
- Werken bij Tools4ever
Farid’s Reis bij Tools4ever: Van Consultant naar Manager
9 oktober 2024
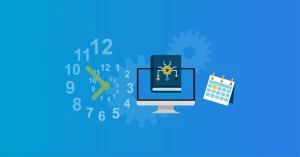
- Product en technologie
Service Automation – Scheduled Tasks
7 oktober 2024
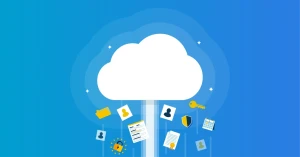
- Industrie en trends
Cloudbeleid overheid in 2025 op de schop?
26 september 2024

- Product en technologie
Slimme RBAC: voorkom rollenexplosie
3 september 2024

- Industrie en trends
Flexwerkers inhuren zonder stress: Tips voor de vakantieperiode
1 augustus 2024
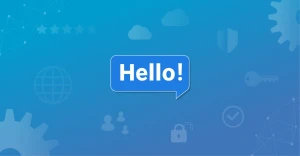
- Werken bij Tools4ever
Ontdek het Verhaal van Anne Merel bij Tools4ever
30 juli 2024